Tips and Tricks
Always set functions' memory, timeout, and log retention.
Otherwise, you will get defaults, which may not be best for your specific case.
Limited log retention will save you costs for storing old Lambda logs that you won't look at anyway
provider:
# common for all functions
memorySize: 512
timeout: 10
logRetentionInDays: 14 ## allowed values: 1, 3, 5, 7, 14, 30, 60, 90, 120, 150, 180, 365, ....
functions:
firstFunction:
handler: index.handler
# function-specific
memorySize: 512
enable HTTP keep-alive
Read here on what this means.
environment:
AWS_NODEJS_CONNECTION_REUSE_ENABLED: 1
Package functions individually
Package functions into separate bundles to reduce their size and Lambda cold start.
functions:
firstFunction:
handler: index.firstHandler
secondFunction:
handler: index.secondHandler
email:
package:
individually: true
Here are useful resources for cold starts, tuning lambdas, and faster functions
Don't import whole SDKs when not needed to reduce function size
Do not import the whole AWS SDK like so:
import {CloudFront, Lambda} from 'aws-sdk'
Instead, do it like this:
import CloudFront from 'aws-sdk/clients/cloudfront'
import Lambda from 'aws-sdk/clients/lambda'
Override generated resource parameters if needed.
Core Serverless Framework (SF) and various plugins reduce boilerplate by generating some resources for us. It's no magic, just CloudFormation resources.
For example, until SF supported IAM auth for HTTP API, we could configure it by ourselves.
functions:
firstFunction:
handler: index.firstHandler
events:
-httpApi:
path: /
method: GET
resources:
extensions:
HttpApiRouteGet:
Properties:
AuthorizationType: AWS_IAM
Set variable values depending on the stage.
You can use a config directory with the structure:
app
├── config
│ └── config.dev.json
│ └── config.staging.json
│ └── config.production.json
└── serverless.yml
custom:
bucketName: ${file(config/config.${self:custom.currentStage}.json):BUCKET_NAME}
provider:
environment:
LOG_LEVEL: ${file(config/config.${self:custom.currentStage}.json):LOG_LEVEL}
Provider/function level variables
Set common environment variables at the provider level, and function-specific variables at the function level. They will be merged together and all will be available in the Lambda runtime.
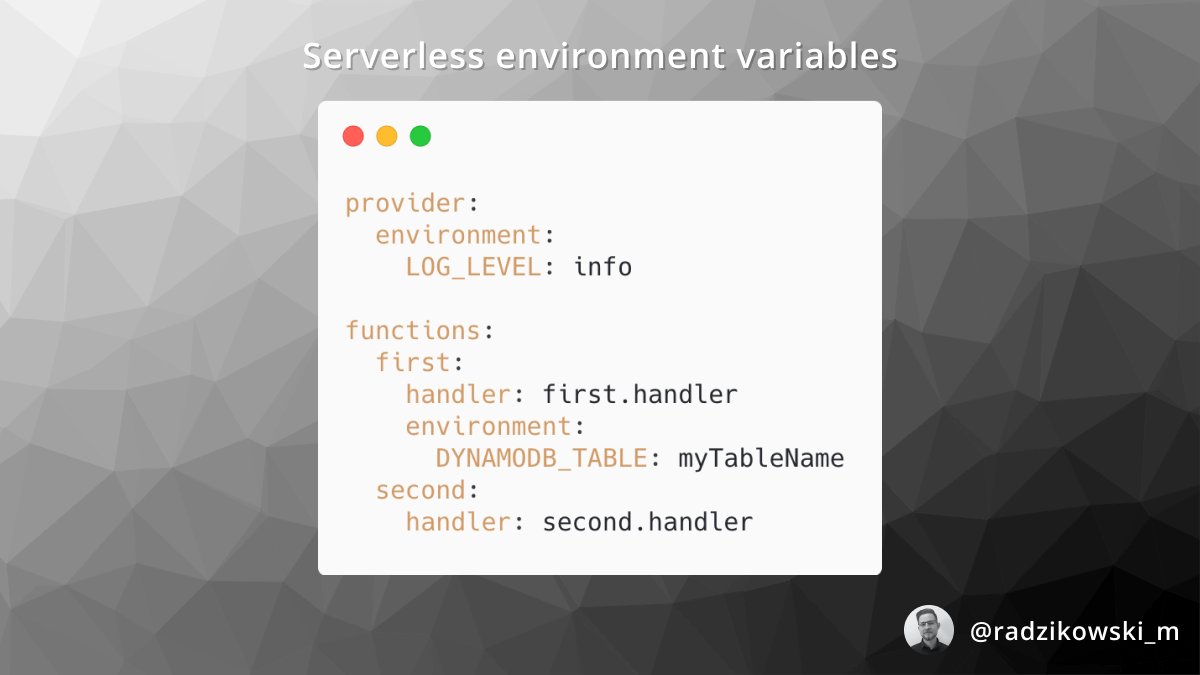
Re-use deployment bucket
If having a project consisting of multiple stacks, reuse a single deployment bucket to limit the number of S3 buckets (and how often you need to request buckets number quota increased).
provider:
deploymentBucket:
name: com.serverless.${self:app}.${self:provider.region}.${self:custom.currentStage}.deploys
Disable Lambda versioning
If not using Lambda versions for rollback etc., disable them. Otherwise, use a plugin to keep only X latest versions.
This will reduce used storage, as it's easier to hit the (default) 75 GB Lambda total size quota than you think.
provider:
versionFunctions: false
Use CloudFormation resource names
Let CloudFormation generate resource names for you. In most cases, it will start with the stack name, with a random suffix.
It will result in consistent, easily identifiable, and unique names. And sometimes not specifying the name blocks some CF updates.
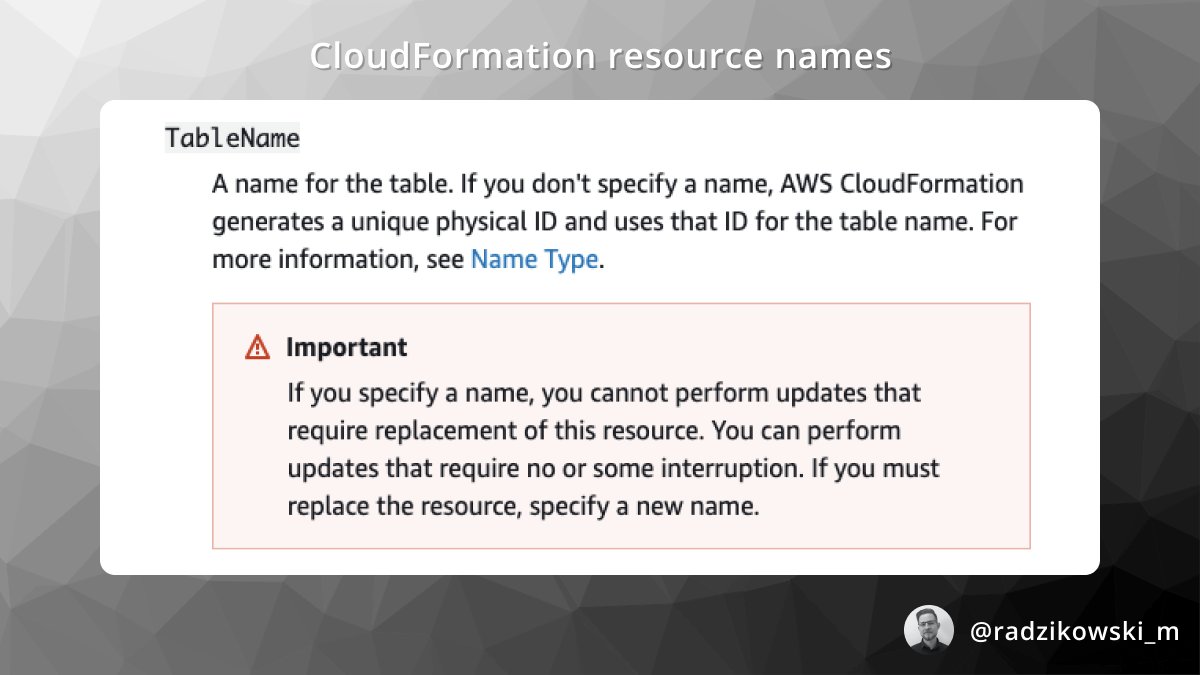