Git & CLI Basics
Get started
Make a directory, then make a local git repository
1. Make new directory
mkdir {repo-name}
3. Create local repository
git init
2. Open new directory
cd {repo-name}
Connect local repo to GitHub
Now, associate your new repository to the relevant GitHub
3. Connect GitHub repo
What this step does is "connect" the remote repository on GitHub to the working directory you created.
git remote add origin {remote-repo-url}
4. Pull the origin main branch
This step pulls all of the files from the remote GitHub repository directory to your working directory.
git pull origin main
Create a branch
The main
branch contains the latest codes and when you make changes, you want to ALWAYS make them on a separate branch so it doesn't impact the most recent working files.
Create your own working branch! Avoid working directly on the main branch. Now, you are ready to make changes.
5. Create new branch
This step is actually two steps in one. First you are breating a branch with the -b
, and at the same time you are switching to that branch with checkout
.
git checkout -b feature_branch_name
If you don't want behavior where changes "travel with you" to a different branch, you should use:
git switch branch_name
Submit your changes
Once you make changes to the repository on your local branch, you are ready to commit your files and push the files to the remote repository. We do this in 3 steps: 1. add all of changes to files you want to commit, 2. then commit those changes, and 3. push the commit to the remote repository.
6. Add files to origin branch
git add {files}
Push to GitHub
Push to your working branch to GitHub, and submit a pull request for a team member to review before it is merged to main.
7. Commit to origin branch
git commit -m “Add change comments”
8. Push to GitHub branch
git push -u origin branch_name
Merge branch to origin
Make sure you are in main and your changes in the branch are committed.
Note: If you want to merge, you have to pull the main, and then merge the changes from the branch-name
Merge to origin branch
git merge <branch-name>
Additional Commands
Here are some additional commands for using Git.
Push to GitHub origin main
git push -u origin main
View all branches
git branch -a
View remote branches
git branch -r
Remote branches only.
Check on status
git status
Remote branches only.
View local branches
git branch -l
or git branch
Switch branches
git checkout <branch-name>
Checkout a pull request
gh pr checkout [request#]
Showing others commits in branch
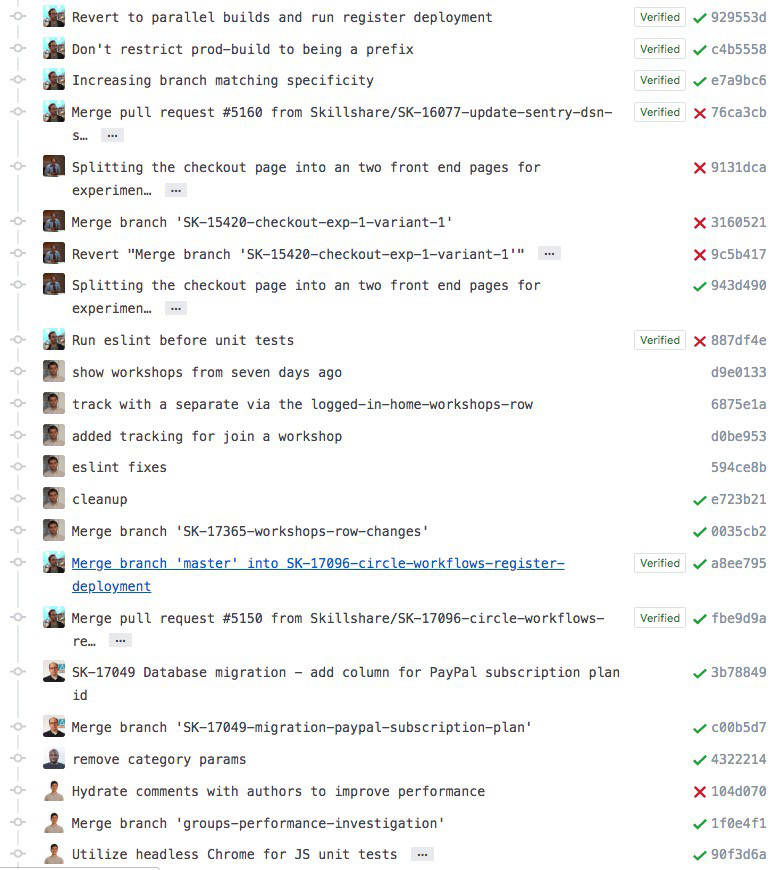
Definitely, you do not want to merge other people’s commit with yours especially when those commits are already part of the target branch(master) in this case
Solution
let's say you have branches like this:
main
branchfeature
we created this branch from the maindemo
we create this branch from 'feature'Then you always take a pull from the
main
branch and itselffeature
branch in a feature branch and push it.
git checkout feature
git pull origin main
git pull origin feature
git push origin feature
- In the
demo
branch you always take a pull from thefeature
branch and push it.
git pull origin feature