Nomenclature
Conventions
These rules follow Airbnb's methodology.
Acronyms
Acronyms should either be all capitalized or all lowercase.
Note: this rule can only be broken when instantiating a new instance with an acronym at the beginning, i.e. const eDILogic = new EDILogic()
// bad
import EdiLogic from './logic/EdiLogic'
// bad
interface userDto {
// ...
}
// bad
import SmsContainer from './containers/SmsContainer'
// good
import EDILogic from './containers/SMSContainer'
// good
const HTTPRequests = [
// ...
]
// also good
const httpRequests = [
// ...
]
// best
import TextMessageContainer from './containers/TextMessageContainer'
// best
const requests = [
// ...
]
Camel case
Use camel case when naming objects, functions, and instances.
// bad
const OBJEcttsssss = {}
const this_is_my_object = {}
function c() {}
// good
const thisIsMyObject = {}
function thisIsMyFunction() {}
Pascal case
Use pascal case only when naming constructors, interfaces or classes.
// bad
function user(options) {
this.name = options.name
}
const bad = new user({
name: 'nope'
})
// good
class User {
constructor(options) {
this.name = options.name
}
}
const good = new User({
name: 'yup'
})
Folder Structure
Repo is defined using DDD and clean architecture principles. To learn more, refer to our docs.
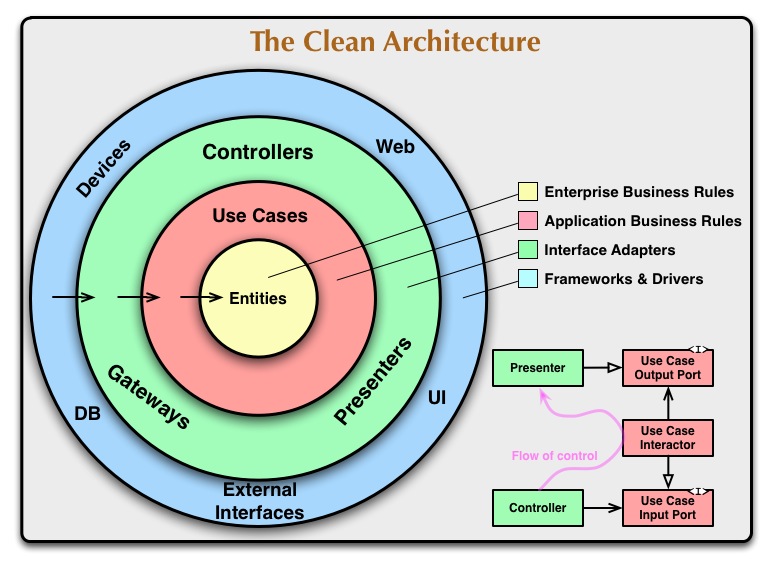
Domain
The domain folder holds the code defining critical core components of the tracking domain. This is the innermost layer in onion architecture, and the code here does not know anything about any of the other folders, logic, or implementation details. As per DDD, this is where the domain entities, repository interfaces and value objects are defined.
Use Cases
The use cases folder contains code that encapsulate the business logic involved in executing the features within our app, and are considered part of the application layer in DDD. They're responsible for retrieving the domain entities in addition to the information that they need in order to execute some domain logic. Use cases can also be executed by other Use Cases from within the application layer as well. Use cases are agnostic of db frameworks, routes and middleware.
Infrastructure
The infra folder contains all of the infrastructure and implementation details of the project including database queries, database configuration, api routes, server details, etc.
Core
The core folder contains common abstract classes.
🧪 Unit and integration tests
Unit and integration tests are written using Jest. To run integration tests, first start the local server (sls offline
), then use the command npm run integration
.
To run unit tests, use the command npm run test
.
To view test coverage, first make sure jest is installed globally yarn global add jest
. Then, use the command jest --coverage
.
Interfaces
One rule of thing to follow with the interface is to start it with a Capital I.